Building Your Mobile Integration
Learn about Finix's mobile in-person payments integrations.
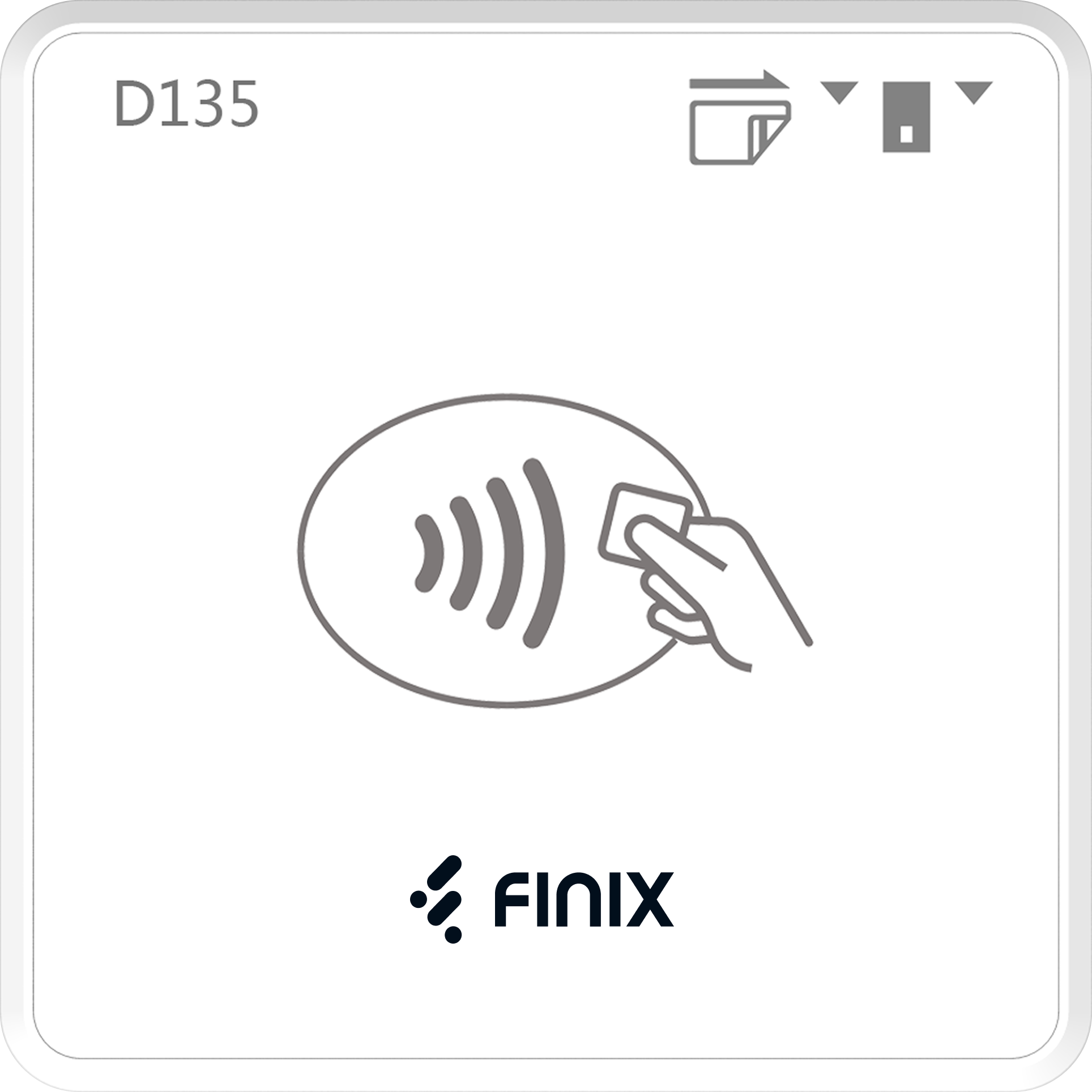
The Pax D135 is a compact, mobile smart card reader designed for modern businesses on the go. Whether you're accepting payments in-store or out in the field, the D135 connects seamlessly to your Android or iOS device via Bluetooth, transforming your phone or tablet into a powerful payment terminal.
For additional details on the Pax D135, see Pax D135 Overview.
Finix offers integration choices for mobile payments:
- Android SDK for Pax D135
- iOS SDK for Pax D135